In this article we are going to cover How to deploy on AWS ECS using GitHub Actions.
Prerequisites:
- An AWS account with ECR (Elastic Container Registry) and ECS (Elastic Container Service) configured.
- A Dockerfile for building your application image.
- An existing GitHub repository for your project.
Step #1:Create an ECR Repository in AWS
Create EC2 Instance, Install AWS CLI and Configure IAM user using AWS CLI, Please follow below article for same
AWS CLI Installation on Linux Servers
Use the AWS CLI or Management Console to create an ECR repository for storing your Docker images.
Syntax:
aws ecr create-repository \
--repository-name MY_ECR_REPOSITORY \
--region MY_AWS_REGION
Example:
aws ecr create-repository \
--repository-name nodejs-app \
--region ap-south-1
Output:
aws ecr create-repository --region ap-south-1 --repository-name nodejs-app
{
"repository": {
"repositoryArn": "arn:aws:ecr:ap-south-1:908198849120:repository/nodejs-app",
"registryId": "908198849120",
"repositoryName": "nodejs-app",
"repositoryUri": "908198849120.dkr.ecr.ap-south-1.amazonaws.com/nodejs-app",
"createdAt": "2024-03-19T08:18:07.614000+00:00",
"imageTagMutability": "MUTABLE",
"imageScanningConfiguration": {
"scanOnPush": false
},
"encryptionConfiguration": {
"encryptionType": "AES256"
}
}
}
ECR repo got created in AWS
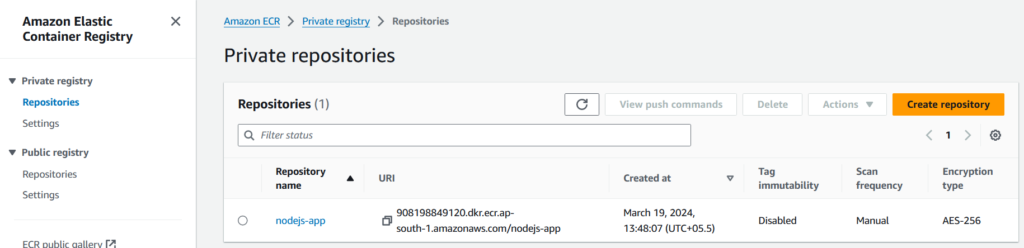
Step #2:Create an ECS Cluster and Task Definition:
Define an ECS cluster in your AWS account where your containerized application will run.
Create a task definition that specifies the container image, CPU, memory, and other configuration details for your application. You can store the task definition as a JSON file in your Git repository.
Step #3:Configure GitHub Secrets
Create secrets in your GitHub repository named AWS_ACCESS_KEY_ID
and AWS_SECRET_ACCESS_KEY
to store your AWS access credentials (ensure least privilege).
Step #4:Create a GitHub Workflow Deploy to AWS ECS
In your GitHub repository, create a .github/workflows
directory and a YAML file defining your workflow.
Here’s a basic structure:
# This workflow uses actions that are not certified by GitHub.
# They are provided by a third-party and are governed by
# separate terms of service, privacy policy, and support
# documentation.
# GitHub recommends pinning actions to a commit SHA.
# To get a newer version, you will need to update the SHA.
# You can also reference a tag or branch, but the action may change without warning.
name: Deploy to Amazon ECS
on:
push:
branches:
- main
env:
AWS_REGION: ap-south-1 # set this to your preferred AWS region, e.g. us-west-1
ECR_REPOSITORY: nodejs-app # set this to your Amazon ECR repository name
ECS_SERVICE: MY_ECS_SERVICE # set this to your Amazon ECS service name
ECS_CLUSTER: MY_ECS_CLUSTER # set this to your Amazon ECS cluster name
ECS_TASK_DEFINITION: MY_ECS_TASK_DEFINITION # set this to the path to your Amazon ECS task definition
# file, e.g. .aws/task-definition.json
CONTAINER_NAME: MY_CONTAINER_NAME # set this to the name of the container in the
# containerDefinitions section of your task definition
jobs:
deploy:
name: Deploy
runs-on: ubuntu-latest
environment: dev
steps:
- name: Checkout
uses: actions/checkout@v4
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@0e613a0980cbf65ed5b322eb7a1e075d28913a83
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: ${{ env.AWS_REGION }}
- name: Login to Amazon ECR
id: login-ecr
uses: aws-actions/amazon-ecr-login@62f4f872db3836360b72999f4b87f1ff13310f3a
- name: Build, tag, and push image to Amazon ECR
id: build-image
env:
ECR_REGISTRY: ${{ steps.login-ecr.outputs.registry }}
IMAGE_TAG: ${{ github.sha }}
run: |
# Build a docker container and
# push it to ECR so that it can
# be deployed to ECS.
docker build -t $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG .
docker push $ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG
echo "image=$ECR_REGISTRY/$ECR_REPOSITORY:$IMAGE_TAG" >> $GITHUB_OUTPUT
- name: Fill in the new image ID in the Amazon ECS task definition
id: task-def
uses: aws-actions/amazon-ecs-render-task-definition@c804dfbdd57f713b6c079302a4c01db7017a36fc
with:
task-definition: ${{ env.ECS_TASK_DEFINITION }}
container-name: ${{ env.CONTAINER_NAME }}
image: ${{ steps.build-image.outputs.image }}
- name: Deploy Amazon ECS task definition
uses: aws-actions/amazon-ecs-deploy-task-definition@df9643053eda01f169e64a0e60233aacca83799a
with:
task-definition: ${{ steps.task-def.outputs.task-definition }}
service: ${{ env.ECS_SERVICE }}
cluster: ${{ env.ECS_CLUSTER }}
wait-for-service-stability: true
Explanation:
- The workflow triggers on a push to the
main
branch. - It checks out the code from the repository.
- The
aws-login
action authenticates with AWS using the stored secrets. - The workflow builds the Docker image using
docker build
. - It pushes the image to your ECR repository using
docker push
. - The final steps (commented as examples) demonstrate using the AWS CLI to
- Register the task definition (replace with the appropriate command for your chosen method).
- Update the service in your ECS cluster to deploy the new image (replace with the appropriate command for your chosen method).
Conclusion:
In this article we have covered How to deploy on AWS ECS using GitHub Actions.
Related Articles:
Deploy to Kubernetes using GitHub Actions
Reference:
Deploying to Amazon Elastic Container Service github actions official page