Similar Posts
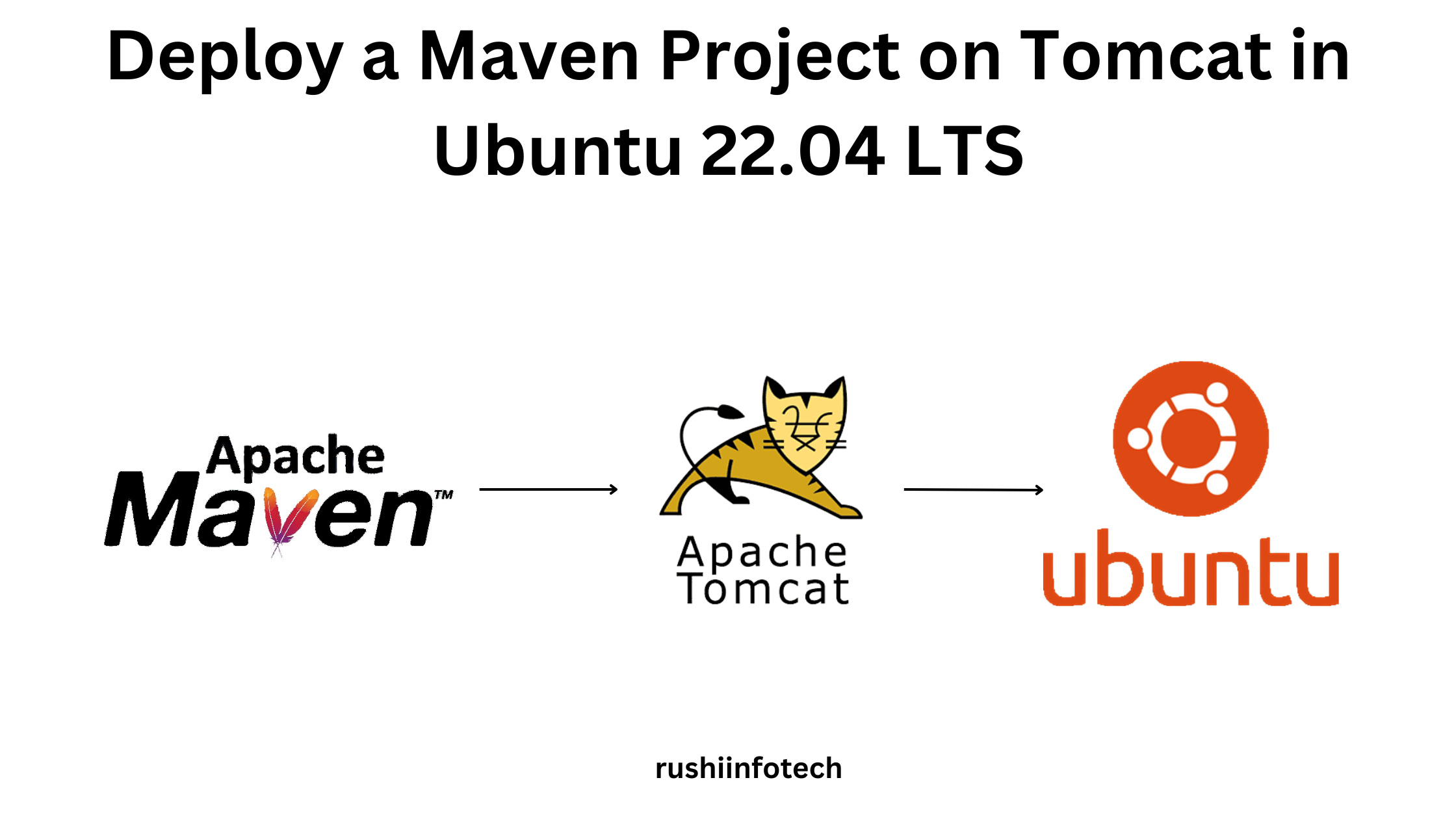
Deploy a Maven project on Tomcat in Ubuntu 22.04 LTS
You need to be logged in to view this content. Please Log In. Not a…
How to Install & Uninstall Git on Linux Ubuntu Os ?
You need to be logged in to view this content. Please Log In. Not a…
Exploring Linux Commands: A Practical Guide with Examples
You need to be logged in to view this content. Please Log In. Not a…
![Java 18 Installation on Ubuntu 22.04 LTS [5 Steps]](https://rushiinfotech.in/wp-content/uploads/2023/09/Java-18-Installation-on-Ubuntu-22.04-LTS.png)
Java 18 Installation on Ubuntu 22.04 LTS [5 Steps]
You need to be logged in to view this content. Please Log In. Not a…

“Discovering Linux: The Heart of Open-Source Computing”
You need to be logged in to view this content. Please Log In. Not a…
What is Linux ?
You need to be logged in to view this content. Please Log In. Not a…