Similar Posts
What’s an Ansible Playbook?
You need to be logged in to view this content. Please Log In. Not a…
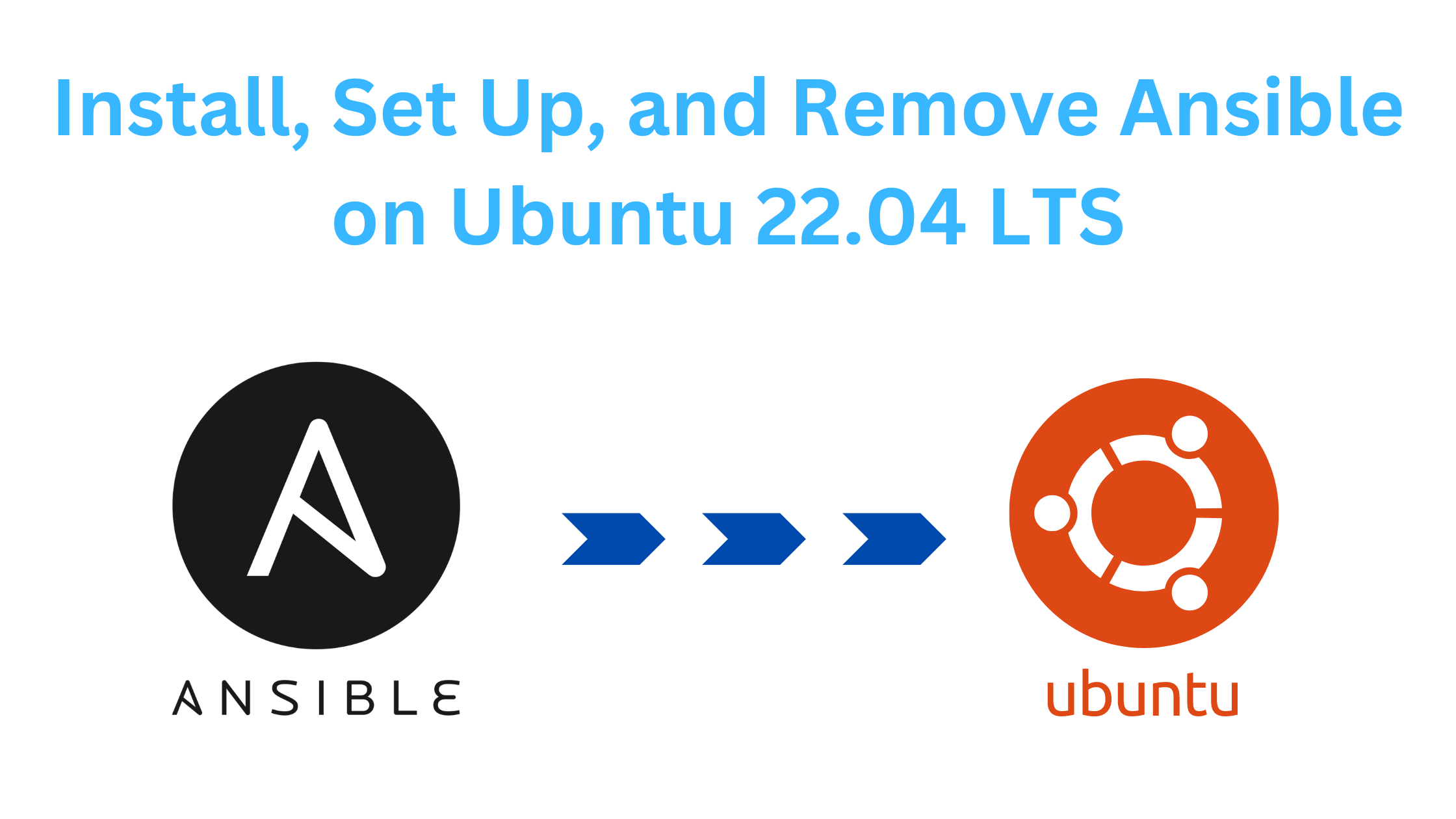
The Easy Way to Install, Set Up, and Remove Ansible on Ubuntu 22.04 LTS: A Beginner’s Guide
You need to be logged in to view this content. Please Log In. Not a…

What is Ansible Vault?
You need to be logged in to view this content. Please Log In. Not a…