Similar Posts

Integrate OpenTelemetry for .NET Application
You need to be logged in to view this content. Please Log In. Not a…
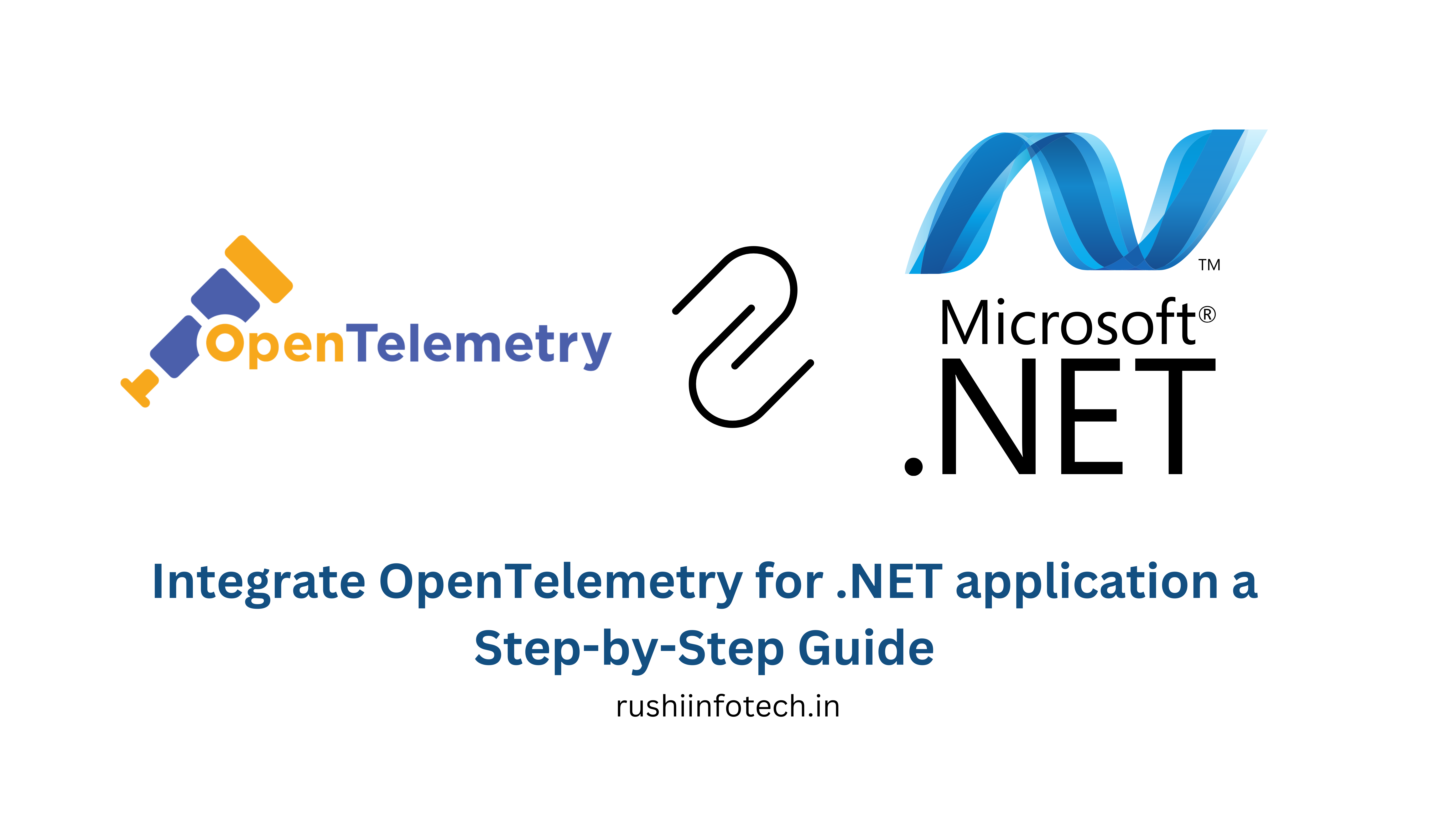
Integrate OpenTelemetry for .NET application a Step-by-Step Guide (4Steps)
You need to be logged in to view this content. Please Log In. Not a…

Instrumentation of .NET Application Metrics Using OpenTelemetry, Prometheus, and Grafana
You need to be logged in to view this content. Please Log In. Not a…

Collect HTTP Metrics for .NET Application Using OpenTelemetry and Prometheus
You need to be logged in to view this content. Please Log In. Not a…