Similar Posts
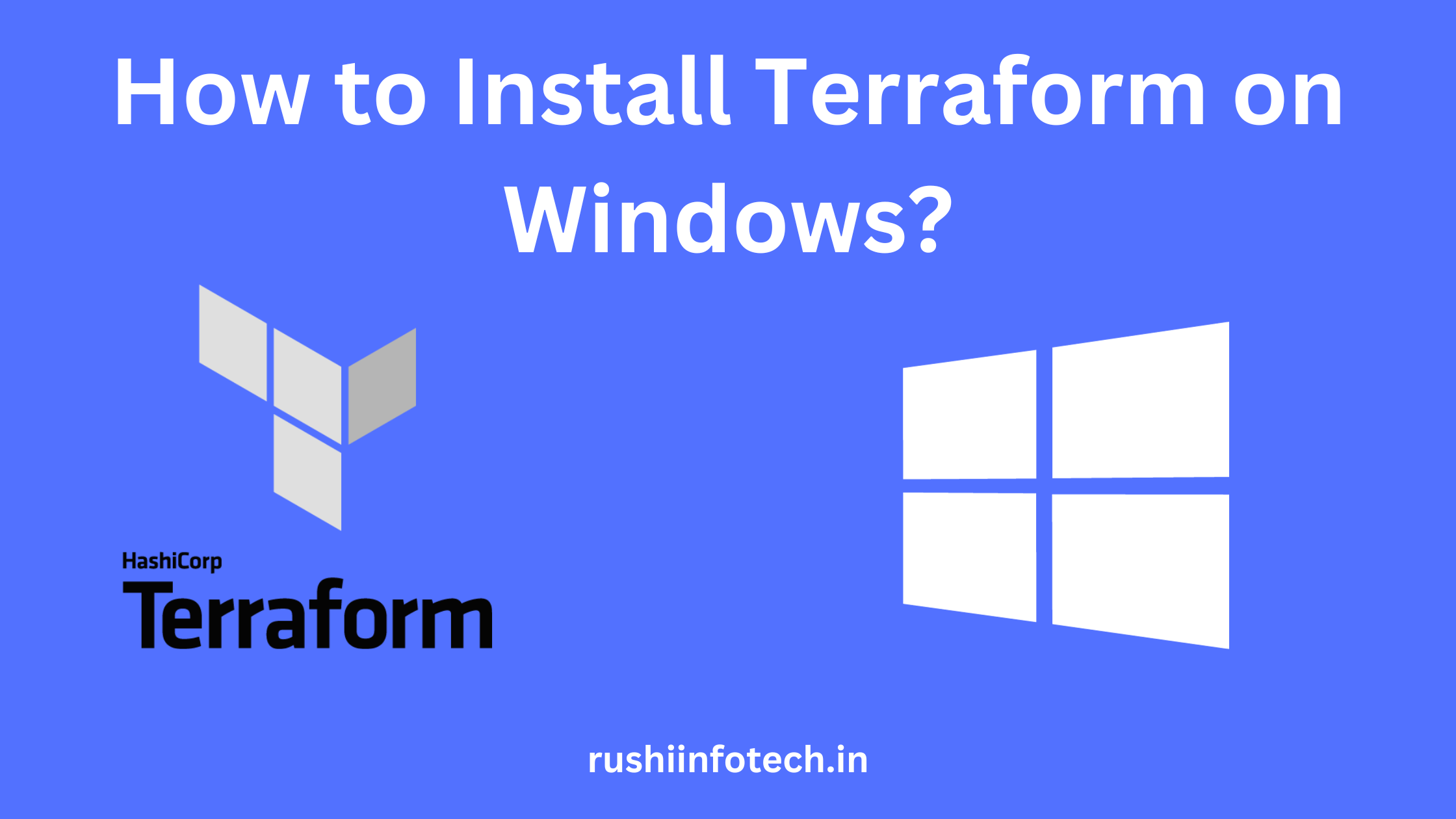
How to Install Terraform on Windows: A Step-by-Step Guide [4 Steps]
You need to be logged in to view this content. Please Log In. Not a…

Creating an EC2 Instance in AWS with Terraform: A Step-by-Step Guide
You need to be logged in to view this content. Please Log In. Not a…
How to Create IAM User through Terraform?
You need to be logged in to view this content. Please Log In. Not a…
What is Terraform?
Introduction In the evolving landscape of digital technology, managing infrastructure effectively is crucial for any…
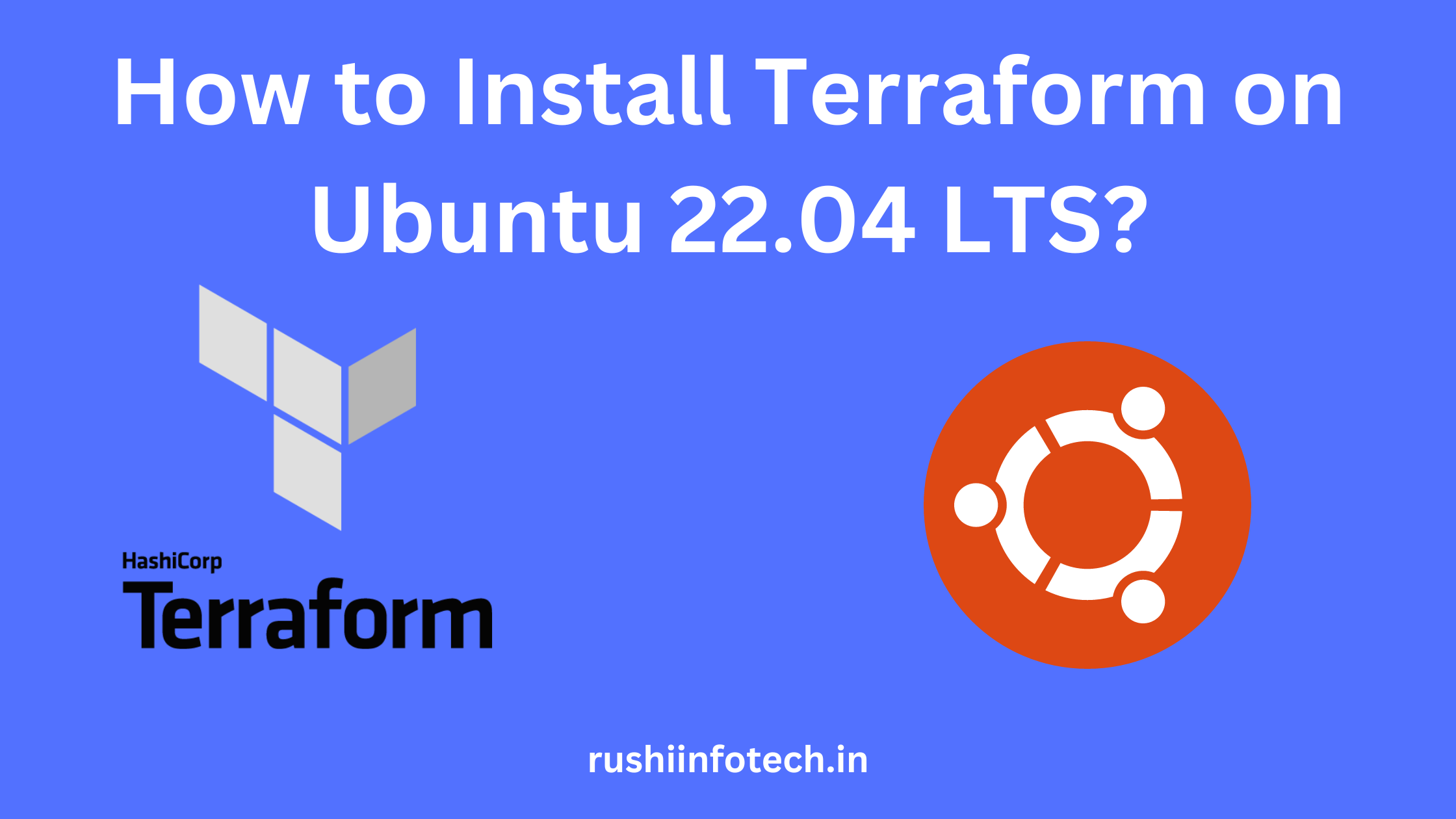
How to Install Terraform on Ubuntu 22.04 LTS?
You need to be logged in to view this content. Please Log In. Not a…
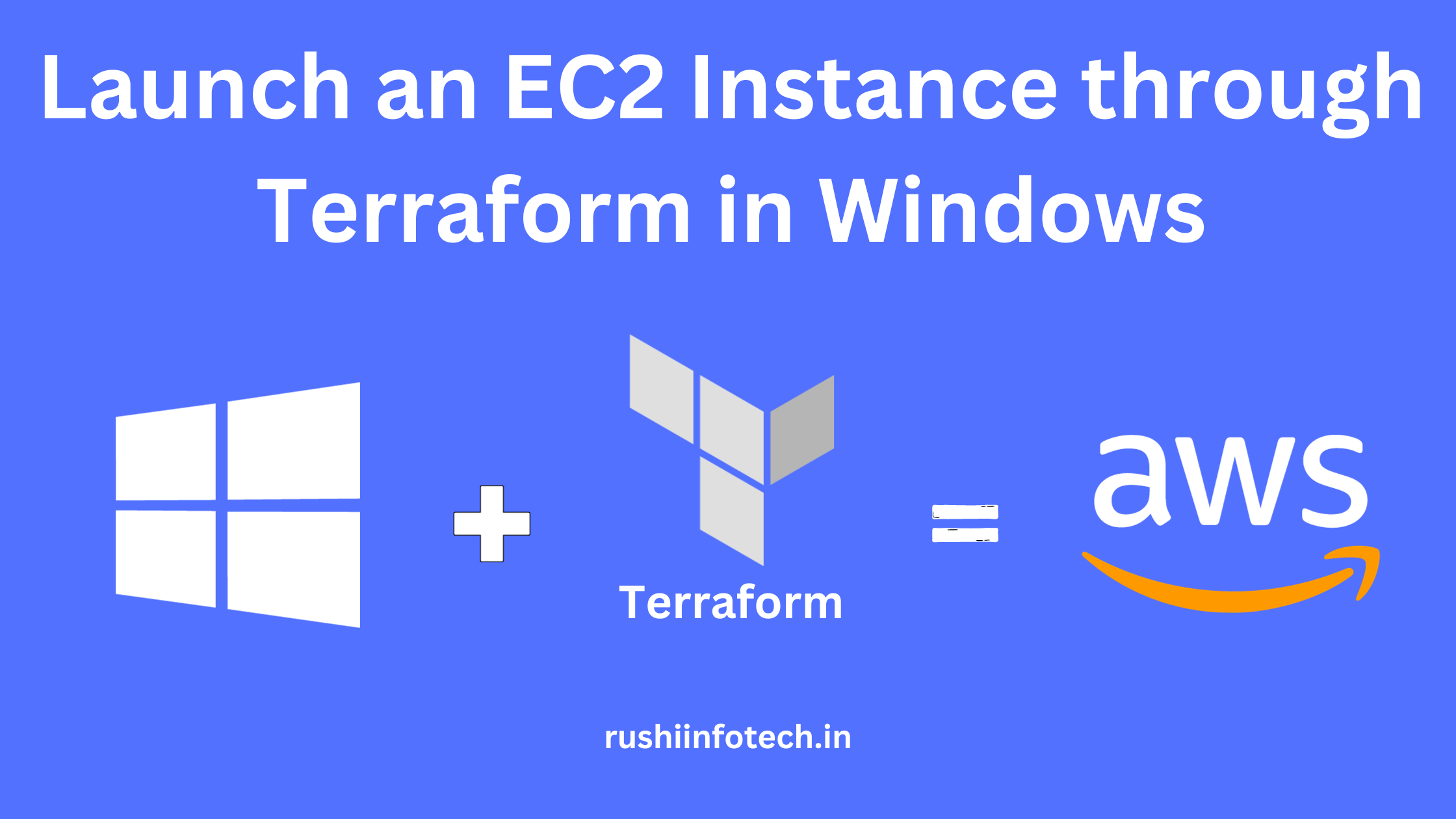
How to Launch EC2 Instance through Terraform?
You need to be logged in to view this content. Please Log In. Not a…